React is a popular Javascript library (which is open-source) for developing user interfaces. It’s an ideal solution for any modern online or mobile app’s view layer.
The best thing is that React works well with various frameworks and tools, allowing it to handle many front-end development tasks.
React, for instance, will enable developers to construct interactive interfaces without reloading the page. This feature creates user interfaces that are aesthetically appealing, intuitive, and performance-driven.
You can create a pie chart in React with FusionCharts, much like various other aesthetically appealing charts.
FusionCharts makes creating dashboards for your online and mobile applications relatively simple. Detailed documentation has made the charts easy to use. Cross-browser compatibility and a standard API add to the features of these charts.
This platform has everything from fundamental charts – like line, column, and pie – to domain-specific charts – like heatmaps, radar, and stock charts. In this article, we will work to show you how to make your own React pie chart.
What Are Pie Charts And Their Advantages?
Charts and graphs are easy methods to illustrate statistical data for organizations, rather than simply providing a series of statistics. The pie chart is the most well-known of them. Its name is derived from its similarity to a pie since it displays data in slices and has a circular form.
A pie chart is a good option when you need to show simple data because it’s easy to make and interpret. Other visualization methods, such as a bar graph, are better for more sophisticated purposes than a pie chart.
At its most basic level, a pie chart is a two-dimensional circle split into a few pieces. The chart as a whole displays the totality of its data; separate slices indicate a proportion of the total. When you take two lines that each account for 50% of turnover, for example, and you design a pie chart to demonstrate product line efficiency, your pie chart will simply have two halves.
Three-dimensional charting, dragging slices, and slice pivoting of charts are some of the most noticeable impacts.
Pie charts are picture representations that are easy to understand. It graphically depicts facts as a portion of a total, which may be an effective way of communicating to even the most inexperienced audience. In addition, pie charts allow the audience to view a quantitative comparison at a glance, allowing them to perform an immediate analysis or swiftly comprehend information.
This graphic representation eliminates readers’ need to analyze or measure underlying statistics. You may edit data in the pie chart to accentuate points you wish to make.
How Can You Make a Pie Chart in React?
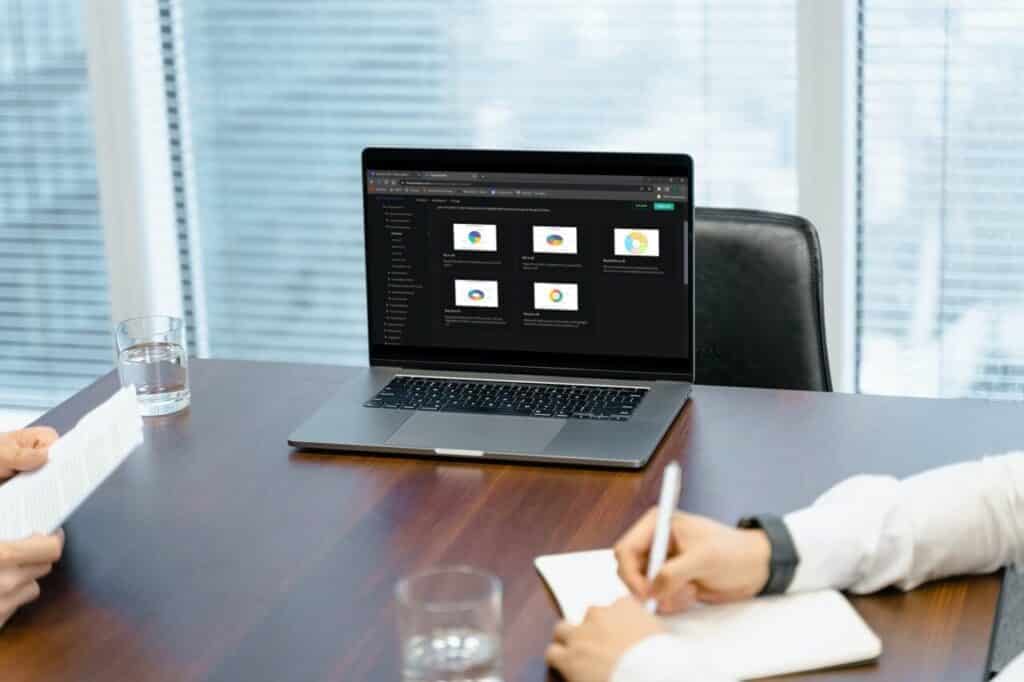
There are different types of pie charts. To see all kinds of charts, click here. We’ll see how to make a few charts using FusionCharts.
2D Pie Chart
A 2D pie chart is a circle divided into segments, each representing a percentage of the total value in a dataset. The graph is excellent for displaying the percentage of elements that make up the whole. The arc length on the graph’s circumference is proportional to the size of the dependent variable.
In a 2D pie chart, the circle’s center connects the arcs using radial lines, splitting the pie into slices. It’s used to indicate the percentage division or sales contribution by product category or brand market share in a specific industry.
Below is an example of how to create a 2D pie chart using React.
import FusionCharts from "fusioncharts";
import charts from "fusioncharts/fusioncharts.charts";
import ReactFusioncharts from "react-fusioncharts";
// Resolves charts dependency
charts(FusionCharts);
const dataSource = {
chart: {
caption: "Market Share of Web Servers",
plottooltext: "<b>$percentValue</b> of web servers run on $label servers",
showlegend: "1",
showpercentvalues: "1",
legendposition: "bottom",
usedataplotcolorforlabels: "1",
theme: "fusion"
},
data: [
{
label: "Apache",
value: "32647479"
},
{
label: "Microsoft",
value: "22100932"
},
{
label: "Zeus",
value: "14376"
},
{
label: "Other",
value: "18674221"
}
]
};
class MyComponent extends React.Component {
render() {
return (
<ReactFusioncharts
type="pie2d"
width="100%"
height="100%"
dataFormat="JSON"
dataSource={dataSource}
/>
);
}
}
3D Pie Chart
A 3D pie chart is a circle partitioned into segments, each representing a percentage of the total value in a dataset. By adding depth to the conventional pie chart, the 3D chart adds additional aesthetics. The graph is excellent for displaying the percentage of elements that make up the whole.
The arc length on the graph’s circumference is proportional to the size of the dependent variable. In a 3D pie chart, the circle’s center connects the arcs using radial lines, splitting the pie into slices.
It indicates the percentage split or contribution of sales by product category or brand market share in a specific industry.
Below is an example of how to create a 3D pie chart using React.
import FusionCharts from "fusioncharts";
import charts from "fusioncharts/fusioncharts.charts";
import ReactFusioncharts from "react-fusioncharts";
// Resolves charts dependancy
charts(FusionCharts);
const dataSource = {
chart: {
caption: "Recommended Portfolio Split",
subcaption: "For a net-worth of $1M",
showvalues: "1",
showpercentintooltip: "0",
numberprefix: "$",
enablemultislicing: "1",
theme: "fusion"
},
data: [
{
label: "Equity",
value: "300000"
},
{
label: "Debt",
value: "230000"
},
{
label: "Bullion",
value: "180000"
},
{
label: "Real-estate",
value: "270000"
},
{
label: "Insurance",
value: "20000"
}
]
};
class MyComponent extends React.Component {
render() {
return (
<ReactFusioncharts
type="pie3d"
width="100%"
height="100%"
dataFormat="JSON"
dataSource={dataSource}
/>
);
}
}
3D Donut Chart
A 3D donut chart is excellent for displaying the proportion of elements in a larger picture. By slicing off doughnut segments, users may additionally highlight data points. The arc length on the graph’s circumference is also proportional to the size of the dependent variable.
It demonstrates the composition of data that contributes to a total of 100 percent. Visualizing the population split into age groups and the proportion of market segments to the bottom line, are examples.
Below is an example of how to create a Donut 3D pie chart using React.
import FusionCharts from "fusioncharts";
import charts from "fusioncharts/fusioncharts.charts";
import ReactFusioncharts from "react-fusioncharts";
// Resolves charts dependancy
charts(FusionCharts);
const dataSource = {
chart: {
caption: "Top 5 countries with Global Oil Reserves",
subcaption: "MMbbl= One Million barrels",
enablesmartlabels: "1",
showlabels: "1",
numbersuffix: " MMbbl",
usedataplotcolorforlabels: "1",
plottooltext: "$label, <b>$value</b> MMbbl",
theme: "fusion"
},
data: [
{
label: "Venezuela",
value: "290"
},
{
label: "Saudi",
value: "260"
},
{
label: "Canada",
value: "180"
},
{
label: "Iran",
value: "140"
},
{
label: "Russia",
value: "115"
}
]
};
class MyComponent extends React.Component {
render() {
return (
<ReactFusioncharts
type="doughnut3d"
width="100%"
height="100%"
dataFormat="JSON"
dataSource={dataSource}
/>
);
}
}
What Are the Benefits of Using FusionCharts to Make Pie Charts in React?
In conclusion, FusionCharts makes creating React graphs simple. It is a comprehensive JavaScript framework for interactive and responsive charts.
In FusionCharts it is easy to integrate into your online application, requiring only a few lines of code. This platform also provides several graph examples, all of which come with source code and are updated and bug-free on a regular basis.
It also offers individual assistance to help you swiftly resolve technical difficulties and details about different charts and dashboards to help you comprehend all the capabilities. FusionCharts also includes a variety of graphs to assist you in successfully visualizing your data.